Checking if an input is empty with CSS
Is it possible to know if an input is empty with only CSS?
I had that question when I tried to make an autocomplete component for Learn JavaScript. Basically, I wanted to:
- Hide a dropdown if the input is empty
- Show the dropdown if the input is filled
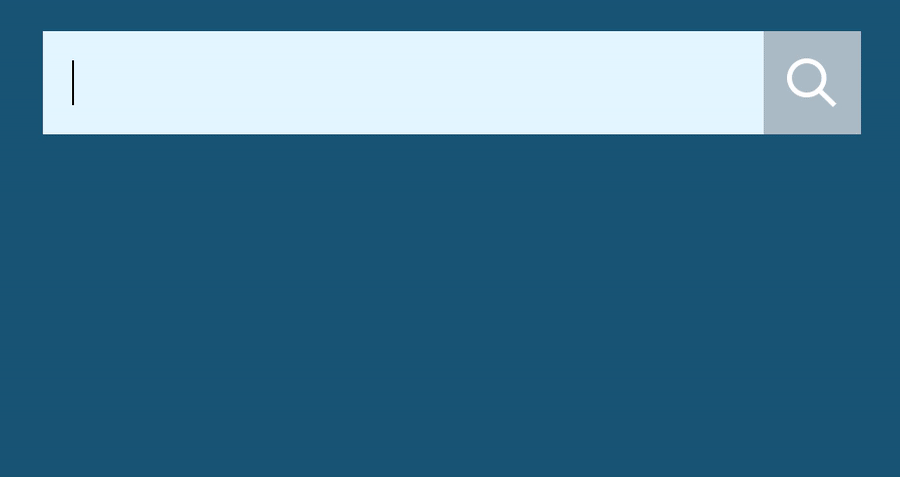
I found a way to do it. It’s not perfect. There are a few nuances involved, but I want to share it with you.
The form
First, let’s build a form so we’re on the same page. We’re going to use a simple form with one input.
<form>
<label for="input"> Input </label>
<input type="text" id="input" />
</form>
When the input is filled, we want to change its border-color
to green. Here’s an example of what we’re creating:
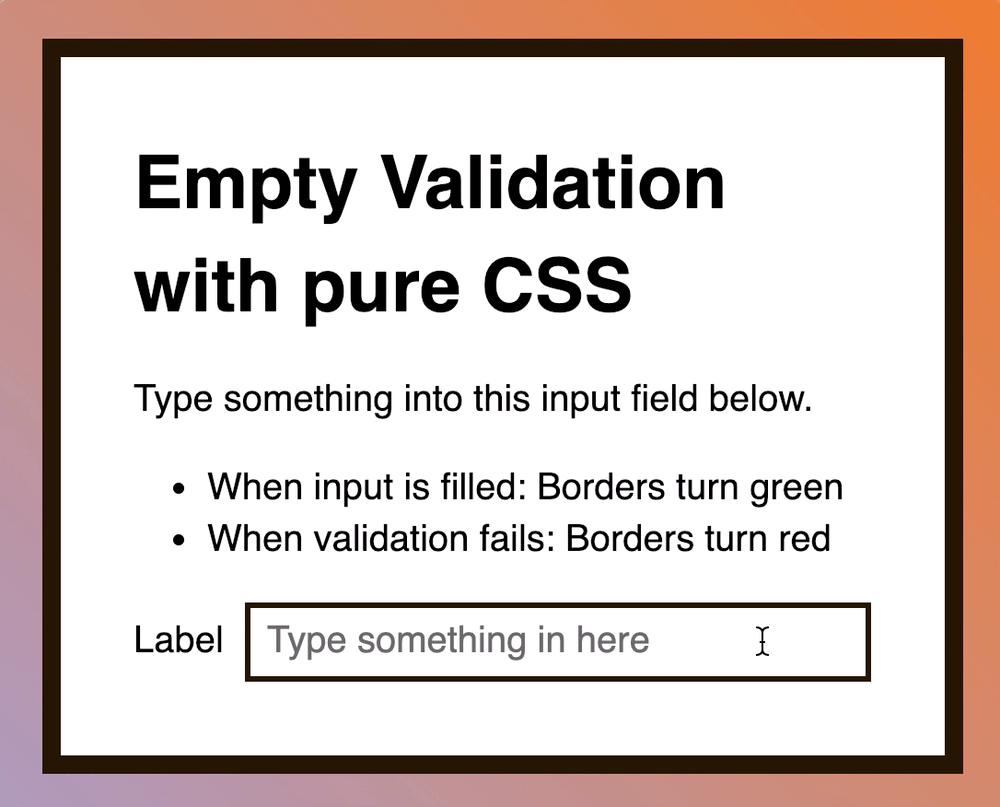
Checking if the input is empty
I relied on HTML form validation to check whether the input is empty. That meant I needed a required
attribute.
<form>
<label> Input </label>
<input type="text" name="input" id="input" required />
</form>
At this point, it works fine when the input is filled. Borders turned green.
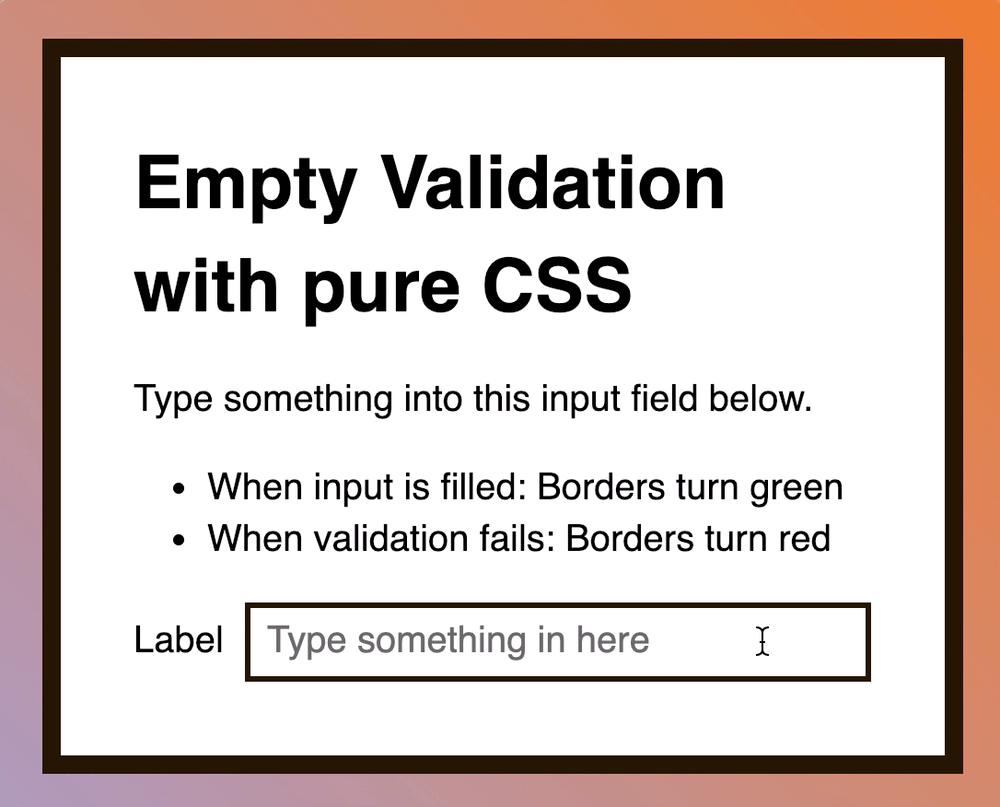
But there’s a problem: If the user enters a whitespace into the field, the borders turn green too.
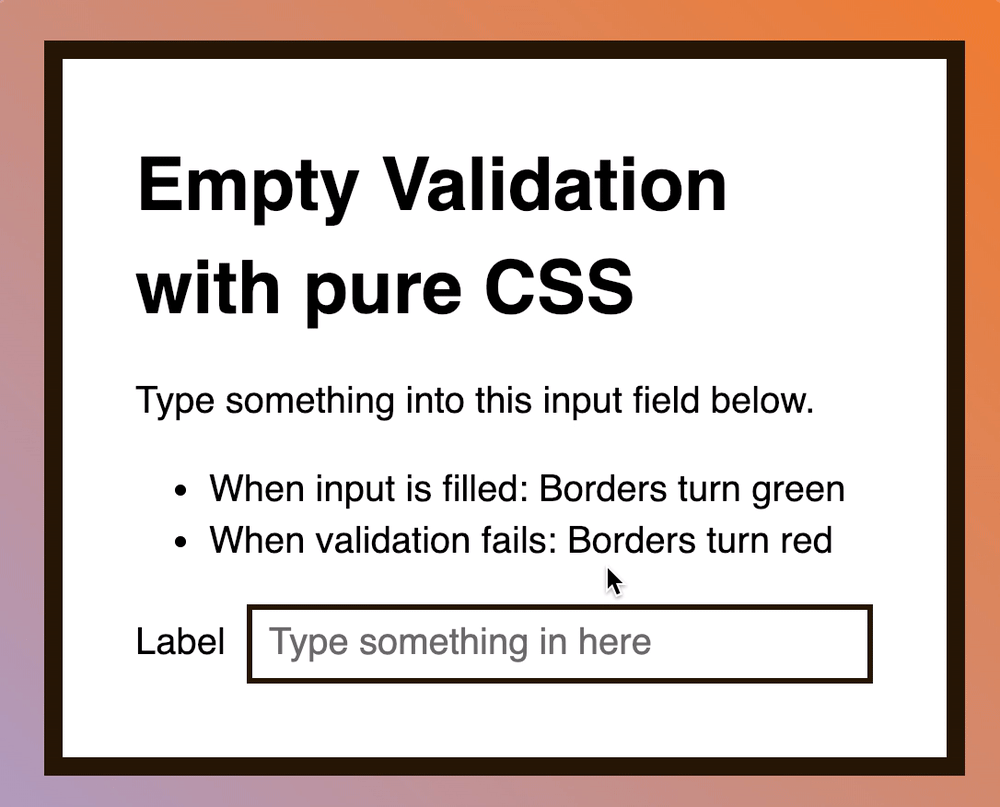
Technically, this is correct. The input is filled because the user typed something into it.
But I didn’t want whitespaces to trigger a blank dropdown menu (for the autocomplete component).
It wasn’t enough. I needed a more stringent check.
Further checks
HTML gives you the ability to validate inputs with regular expressions with the pattern
attribute. I decided to test it out.
Since I didn’t want whitespaces to be recognized, I started with the \S+
pattern. This pattern meant: One or more characters that’s not a whitespace.
<form>
<label> Input </label>
<input type="text" name="input" id="input" required pattern="\S+" />
</form>
Sure enough, it worked. If a user enters a whitespace into the field, the input doesn’t get validated.
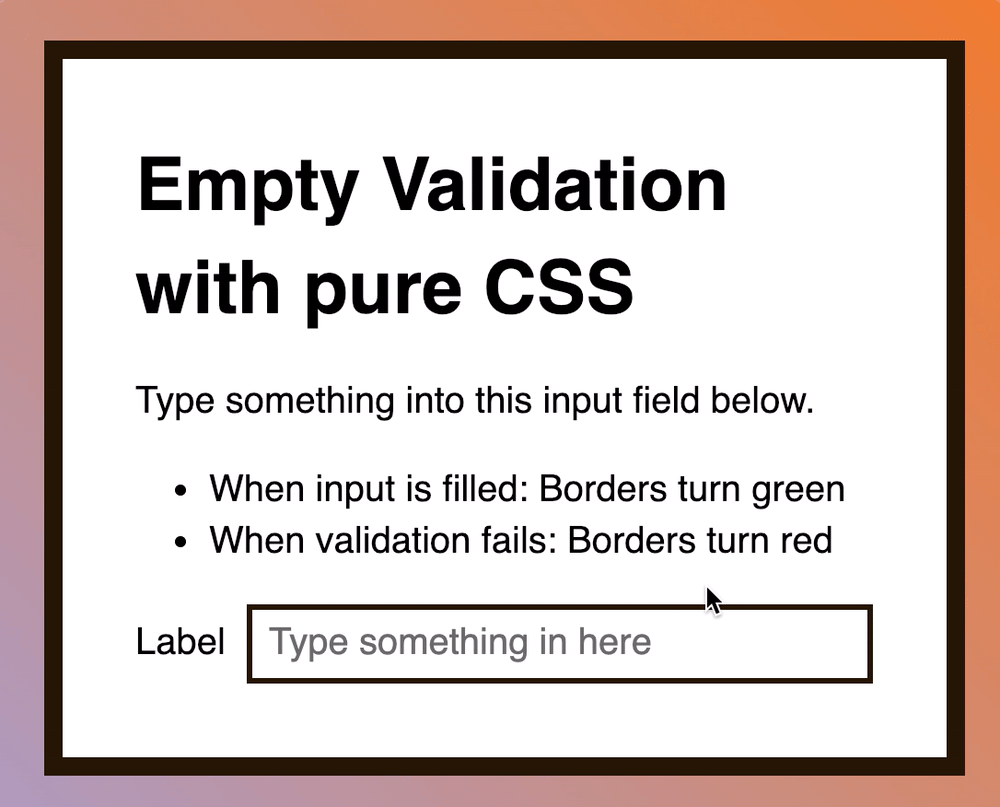
But when a whitespace is entered (anywhere) into the input, the input gets invalidated.
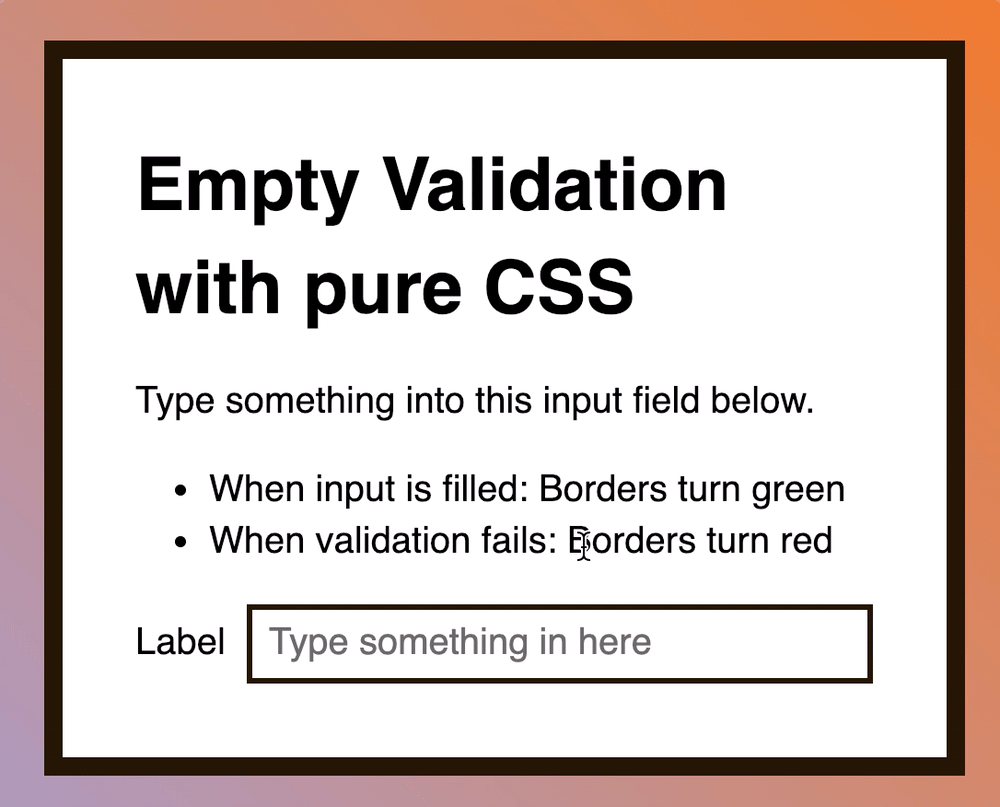
Unfortunately, this pattern didn’t work in my use case.
In Learn JavaScript’s autocomplete component, I taught students how to complete a list of countries. The names of some countries had spaces…
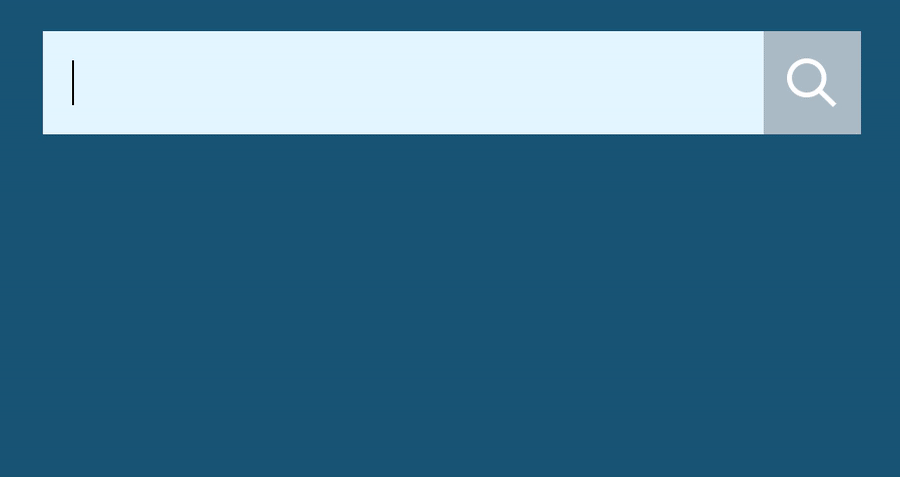
I had to include whitespaces in the mix.
The next best alternative I could think of is \S+.*
. This means 1 or more non-whitespace characters, followed by zero or more (any) characters.
<form>
<label> Input </label>
<input type="text" name="input" id="input" required pattern="\S+.*" />
</form>
This worked! I can enter whitespaces into the mix now!
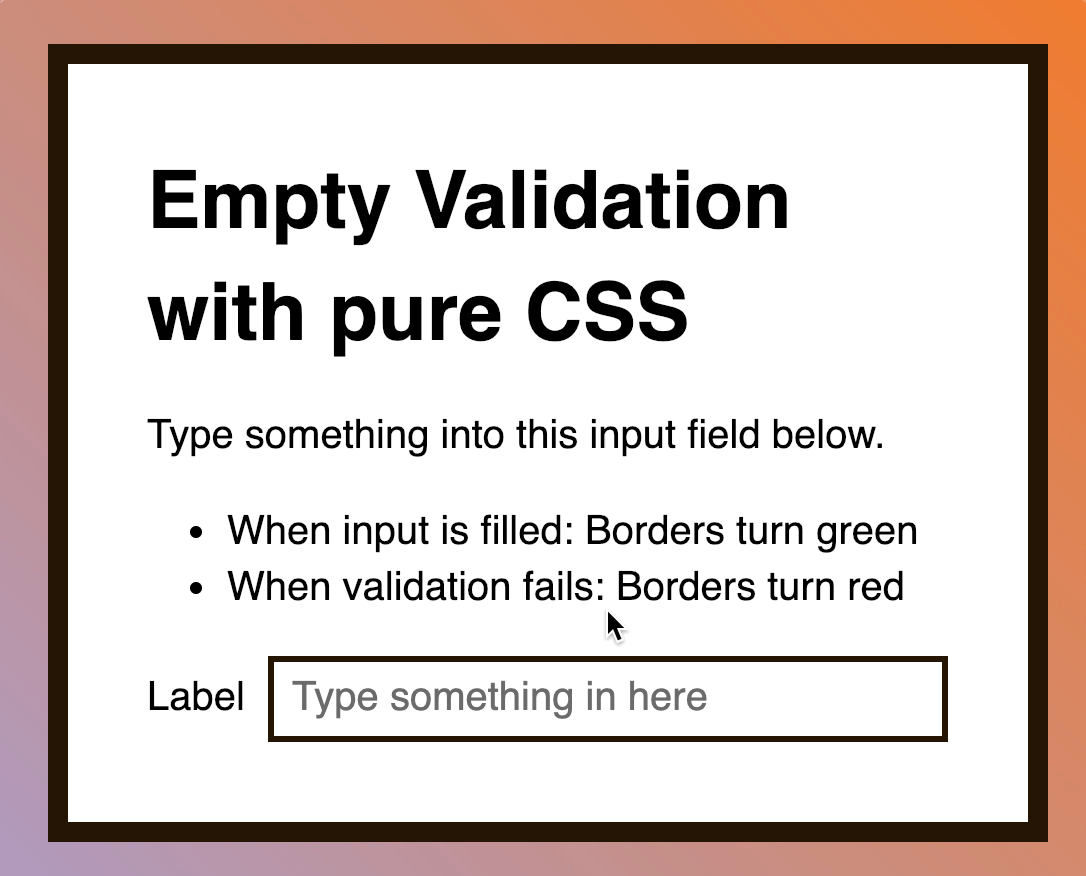
But there’s one more problem… the input doesn’t validate if you START with a whitespace…
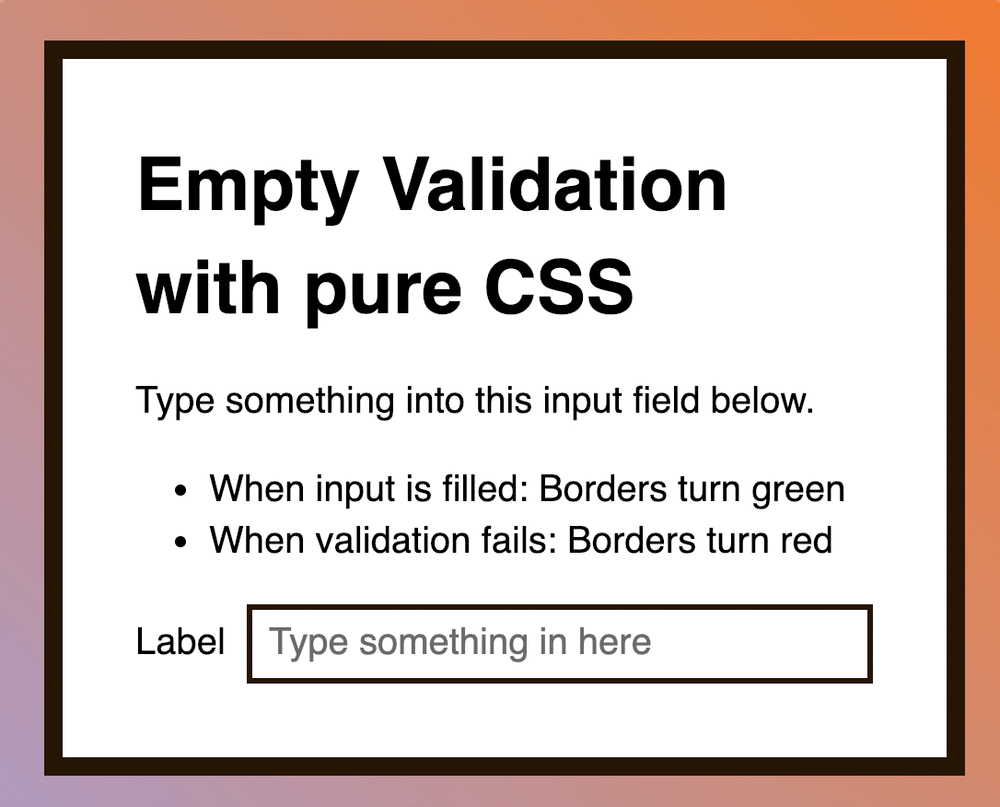
And that’s the problem I couldn’t resolve. More on this later.
When I worked on this article, I came across another interesting question: Is it possible to style an invalid state when the input is filled incorrectly?
Invalidating the input
We don’t want to use :invalid
because we’ll kickstart the input with an invalid state. (When the input is empty, it’s already invalid).
This is where Chris Coyier swooped in to the rescue with ” Form Validation UX in HTML and CSS“.
In the article, Chris talks about a :placeholder-shown
pseudo-class. It can be used to check whether a placeholder is shown.
The idea is:
- You add a placeholder to your input
- If the placeholder is hidden, it means the user typed something into the field
- Proceed with validation (or invalidation)
Here’s the CSS (simplified version. For the complete version, check out Chris’s article)
/* Show red borders when filled, but invalid */
input:not(:placeholder-shown) {
border-color: hsl(0, 76%, 50%);
}
Since I had both validation AND invalidation styles, I had to ensure the valid styles came after the invalid styles.
/* Show red borders when filled, but invalid */
input:not(:placeholder-shown) {
border-color: hsl(0, 76%, 50%);
}
/* Show green borders when valid */
input:valid {
border-color: hsl(120, 76%, 50%);
}
Here’s a demo for you to play with:
See the Pen Pure CSS Empty validation by Zell Liew (@zellwk) on CodePen.
Note: Edge doesn’t support :placeholder-shown
, so it’s probably not a good idea to use it in production yet. There’s no good way to detect this feature.
Now back to the problem I couldn’t resolve.
The problem with pattern
The pattern
attribute is wonderful because it lets you accept a regular expression. This regular expression lets you validate the input with anything you can think of.
But… the regular expression must match the text completely. If the text doesn’t get matched completely, the input gets invalidated.
This created the problem I mentioned above. (Reminder of the problem: If a user enters a whitespace first, the input becomes invalid).
I couldn’t find a regular expression that worked for all use-cases that I thought of. If you want to try your hand at creating a regular expression that I need, I’d be more than welcome to receive the help!
Here are the use-cases:
// Should not match
''
' '
' '
' '
// Should match
'one-word'
'one-word '
' one-word'
' one-word '
'one phrase with whitespace'
'one phrase with whitespace '
' one phrase with whitespace'
' one phrase with whitespace '
(Then again, I might be overthinking it… 🙄).
Update: Problem solved!
Many readers were generous enough to email me their solutions. I want to thank everyone who helped. Thank you so much!
The cleanest solution I received is: .*\S.*
by Daniel O’Connor. This means:
.*
: Any character\S
: Followed one non-whitespace character.*
: Followed by any character
Other regexes I received include:
.*\S+.*
by Matt Mink.\s*\S.*
by Sungbin Jo^\s?(?=\S).*
with a lookahead by Konstantin
And many others!
Here’s a codepen with the updated solution by Daniel:
See the Pen Pure CSS Empty validation by Zell Liew (@zellwk) on CodePen.
Wrapping up
Yes, it is possible to validate a form with pure CSS, but there are potential problems with validation when whitespace characters are involved.
If you don’t mind the whitespaces, it works perfectly. Have fun trying this pattern out! (Sorry, I can’t help it).