The difference between HTML, CSS, and JavaScript
In this article, I want to explain the difference between HTML, CSS, and JavaScript with an analogy. I hope it helps you understand what these languages are, and what they do.
Let’s start with HTML.
HTML
HTML stands for Hypertext Markup Language. It creates the structure of a website.
Let’s use a house as an analogy. Think about the house you currently live in. How many rooms does it have? My house has:
- One living room
- One kitchen
- Two bedrooms
If I write this structure into code, it might look something like this:
<house>
<living-room></living-room>
<kitchen></kitchen>
<bedroom></bedroom>
<bedroom></bedroom>
</house>
Each item within angled brackets (<
and >
) is called a tag. Here, <house>
is a tag. It has one <living-room>
, one <kitchen>
, and two <bedroom>
s in it.
HTML is similar to the code above. But HTML requires you to use a predefined list of tags instead of <house>
and <living-room>
. You can find a list of all possible tags on MDN. Examples of these tags include:
<div>
,<section>
,<article>
<h1>
,<h2>
,<h3>
,<h4>
,<h5>
,<h6>
,<p>
,<a>
<span>
- …
The most basic of all tags in HTML is <div>
. For this article, I’ll use <div>
to show you what basic HTML is about.
In HTML, we can’t write <house>
because <house>
is not a valid HTML tag. We can use <div>
as a replacement for the <house>
tag. But we need some way to identify “house”.
To identify “house”, we use a class. An HTML version of the house looks like this:
<div class="house">
<div class="living-room"></div>
<div class="kitchen"></div>
<div class="bedroom"></div>
<div class="bedroom"></div>
</div>
We can dive deeper.
Consider the room you are in. What furniture do you have in the room? In my room, there’s a chair, a table, a wardrobe, and a bed.
The HTML version of this would be:
<div class="house">
<div class="living-room"></div>
<div class="kitchen"></div>
<div class="bedroom">
<!-- Items in the bedroom -->
<div class="chair"></div>
<div class="table"></div>
<div class="wardrobe"></div>
<div class="bed"></div>
</div>
<div class="bedroom"></div>
</div>
The words between <--
and -->
are called comments. They’re words meant for you and me to read. Browsers will not try to understand what they are. We use comments to write down thoughts for ourselves and other developers.
You can still dive deeper.
On my bed, I have:
- Two pillows
- One bolster
<div class="house">
<div class="living-room"></div>
<div class="kitchen"></div>
<div class="bedroom">
<!-- Items in the bedroom -->
<div class="chair"></div>
<div class="table"></div>
<div class="wardrobe"></div>
<div class="bed">
<!-- Items on my bed -->
<div class="pillow"></div>
<div class="pillow"></div>
<div class="bolster"></div>
</div>
</div>
<div class="bedroom"></div>
</div>
If you go on, you can create the entire structure of your house in HTML. This is what I mean when I say HTML is the structure of a website.
<div class="house">
<div class="living-room"><!-- ... --></div>
<div class="kitchen"><!-- ... --></div>
<div class="bedroom"><!-- ... --></div>
<div class="bedroom"><!-- ... --></div>
</div>
CSS
CSS stands for Cascading Stylesheets. It lets you make a website look nicer. In industry terms, we say you “style” a website with CSS.
You can style an item by writing the following:
selector {
property: value;
}
selector
refers to the tag or class, (or attributes, if you get a bit more advanced) you want to style. property
and value
pairs let you defined the style. These would make more sense if we go back to our house analogy again.
Consider your bedroom. How’s the furniture arranged? For my bedroom, my:
- Bed is placed in the north-eastern corner
- Wardrobe is placed in the south-eastern corner
- door is at the south-west corner
On a website, we don’t have north/east/south/west directions. But we can still say where things are with top/right/bottom/left.
Let’s say my bed is placed in the top-right corner of the room. This is what I might write:
.bed {
position: absolute;
top: 0;
right: 0;
}
This means:
- Set
position
toabsolute
forbed
class elements - Push it all the way to the top
- Push it all the way to the right
Besides locations, you can also change things like size and color.
.bed {
width: 400px;
height: 200px;
background-color: lightskyblue;
}
You can find a list of all CSS properties here.
Note: This is a huge list. You might get overwhelmed if you look at it. The good news is, you don’t have to learn everything at once. If you’re starting out from scratch, I highly suggest reading ”Interneting is hard”. It explains the basics of HTML and CSS in a simple manner.
(I’m working on a step-by-step CSS course to bring you from novice to advance in future. Leave your email here if you want to ask questions/get updates about this course).
JavaScript
HTML and CSS are static. You cannot change HTML and CSS (by changing the HTML or CSS file) after a website gets loaded. But JavaScript gives you the ability to change the HTML and CSS on the page.
Let’s use the analogy of a house again to explain what JavaScript is. By the way, JavaScript and Java are two different things. Don’t mix them up!
Let’s say it’s dark right now. You walk into your room. What would you see?
Nothing. Because it’s pitch black inside.
You move your hands to the switch and you switch on the lights. This is an example of an interaction. Other examples are:
- Turning on the TV
- Turning on the tap for running water
- Turning on the air-conditioner
On a website, a basic interaction is similar to switching on the lights. You click a button and something happens. In this GIF below, I clicked a button and a menu pops out. I clicked the button again and the menu closes.
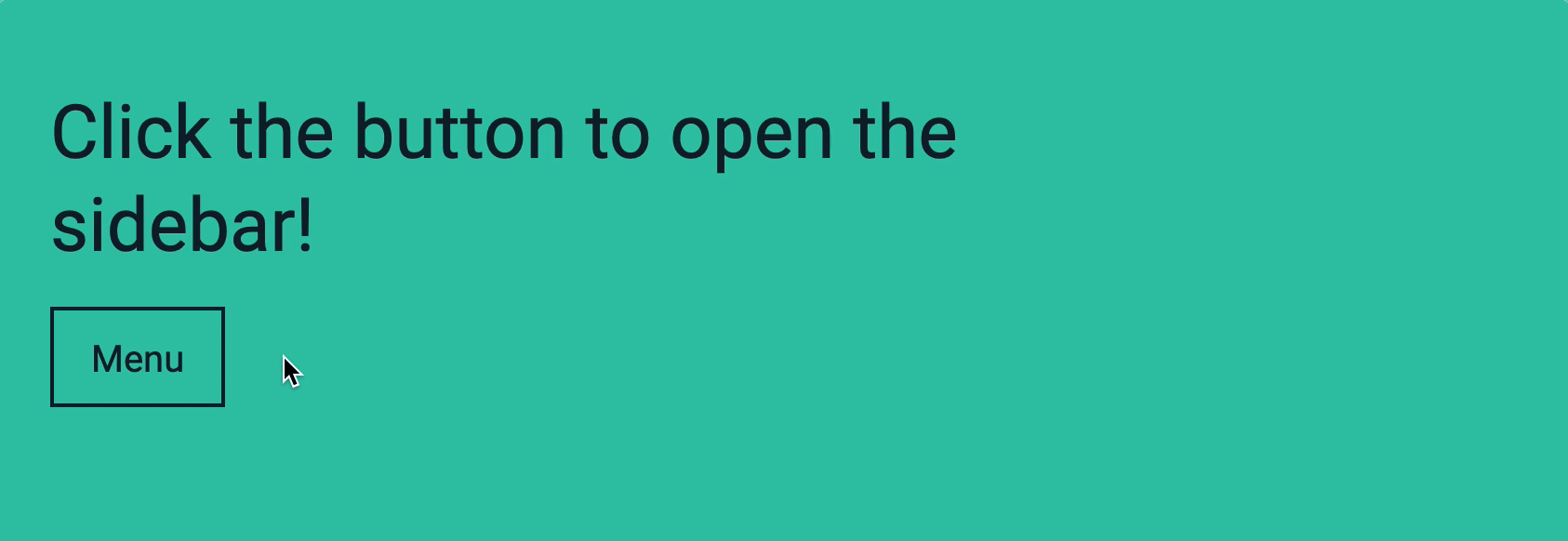
You can do a lot more with JavaScript. Examples are:
- Play an animation when you scroll down
- Open a menu when you hit a button on the keyboard
- Create keyboard shortcuts for your website
- Create a carousel where people can switch between slides
- Make a calculator
There’s so much more about JavaScript than I can say with one blog post. If you’re interested in learning JavaScript, I wrote a course to teach you everything you need to know about JavaScript. It’s called Learn JavaScript. Check it out! You’ll also see some inspiration on what you can build :)
Wrapping up
HTML lets you create the structure of a website.
CSS lets you make the website look nice.
JavaScript lets you change HTML and CSS. Because it lets you change HTML and CSS, it can do tons of things.
That’s it! Hope this helps you better understand the differences between HTML, CSS, and JavaScript!