Setting up Visual Studio Code for Web Development — For Beginners and Intermediate Developers
The coding environment is one of the most important things for a developer.
If you set up your coding environment with the right Linters and Formatters, coding will be very simple. If you don’t, coding will be a nightmare. Why? Because linters and formatters help you catch many errors and speed up the development process (and also, remove a lot of headache you will face from your constant typos)!
Now you know the importance of the environment, the next step is to configure this environment such that it works for you. This is a difficult task because there are so many options available today.
The good news is: You don’t have to do any of that difficult work because I’ve narrowed down the best configuration possible (in my opinion) for you.
I’m going to tell you what the configuration is and how to set it up.
The gist
We’re going to use Prettier, Standard, and ESLint.
- We use Prettier because Prettier is the de-factor code formatter in the industry. Many language formatters build on top of prettier.
- We use Standard because Standard has great JavaScript syntax suggestions — it contains only the essentials (and without any frills).
- We use ESLint because Standard uses it under the hood — and it’s worth a mention because we have to use it for advanced development setup (which I will cover in a future article).
Today, we’re going to talk about a basic setup — one which is super useful for a beginner or intermediate web developers.
When I say beginner or intermediate, I’m assuming you will only use HTML, CSS, and JavaScript in your web development process. You’re not using frameworks like Astro, React, Vue, Svelte, MDX, etc. when you code.
Setting Up Visual Studio Code With Prettier
You will need two VSCode extensions so go ahead and download them:
Using Prettier Standard
Prettier Standard lets you format your code according to both Prettier and Standard. It’s super easy to use.
After you install the extension, you need to set 3 options:
- Set the
defaultFormatter
to this extension. - Remove the VS Code’s default javascript formatter so it doesn’t interfere with Prettier Standard
- Set
formatOnSave
totrue
so VS Code formats your file when you save it.
"editor.defaultFormatter": "numso.prettier-standard-vscode",
"javascript.format.enable": false,
"editor.formatOnSave": true,
When you save your code, the code should now format automatically.
Linting with Standard
Linting code with Standard means you allow Standard to notify you of possible code errors — very much like how you see red squiggly underlines when you misspell a word on your computer. This saves you a lot of time when debugging.

Now, Standard doesn’t lint your code automatically. If you want Standard to lint your, you need to add Standard to your project with npm
. You can do this easily by installing the standard
package.
npm install standard --save-dev
The above assumes you have a package.json
file in your project already. If you don’t have it yet, simply type npm init
and npm will create one for you.
If you don’t know what npm is, npm is a package manager that comes with Node. You can get it by installing Node. Here’s the best way to install and keep Node up to date.
Bonus: Linting both HTML and JavaScript Files
The above setup assumes you only need to lint JavaScript files — which is the common scenario for most beginner and intermediate developers… but there are occasions where you are forced to write JavaScript in HTML, like this:
<body>
<script>
function sayHello(name) {
console.log(`Hello ${name}!`)
}
</script>
</body>
If you need to work with JavaScript in HTML, you cannot lint with Standard. You need to lint with ESLint.
So install the ESLint extension before you continue.
Linting JavaScript Files with ESLint
Like Standard, ESLint doesn’t work automatically so you need to:
- Install ESLint configs and plugins you want to use.
- Include a
.eslintrc
file in your project. - Include the plugins in the
.eslintrc
file.
Since we are using Standard, we can install and use the eslint-config-standard
config.
npm install eslint-config-standard --save-dev
Then we create an .eslintrc
file and the package we just installed as part of our configuration.
// .eslintrc
{
"extends": ["standard"]
}
You should see squiggly red undelines in your JavaScript files at this point.

You cannot use a global .eslintrc
file to make the linter work across all
your projects. I tried 😢. It doesn’t work, because ESLint stopped allowing
plugins in global config files since version 6. At the time of writing, ESLint
is at version 8.
Linting JavaScript in HTML Files with ESLint
If you want to lint JavaScript in HTML files, you can add the eslint-plugin-html
plugin to your project. This enables the linting of JavaScript in the HTML file.
Here’s how you install the plugin:
npm install eslint-plugin-html --save-dev
And how you include the plugin in your .eslintrc
file.
{
"extends": ["standard"],
"plugins": ["html"]
}
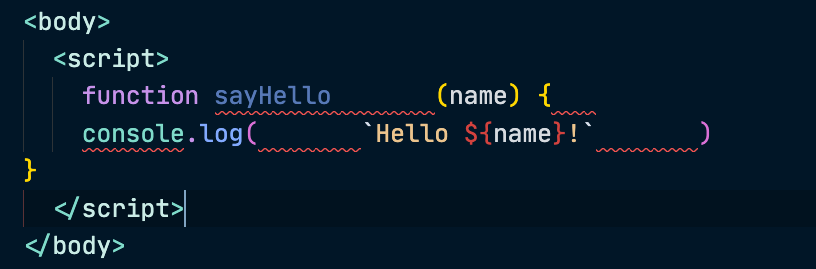
That’s it!
This simple setup will save you hundreds and maybe even thousands of hours as you write code. And this article should have also saved you hours (from googling, trying to set up something nice).
I’ll share more about the advanced setup with Frameworks like Astro, React, Vue, Svelte, MDX, etc. in a future article.